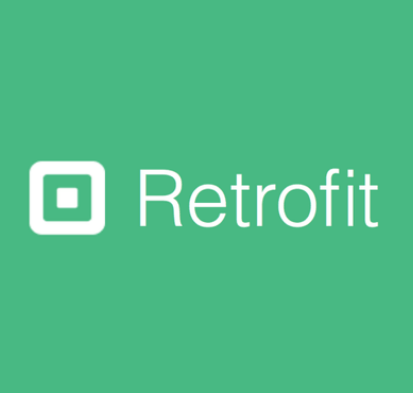
안드로이드 앱을 개발하다보면 외부 서버와의 통신이 필요한 경우가 빈번합니다. 이때 Retrofit 2 라이브러리는 편리하고 효율적인 도구로서 네트워크 통신을 간소화하고 관리할 수 있습니다. 이 글에서는 Retrofit 2를 활용하여 안드로이드 앱에서 서버와의 네트워크 통신을 구현하는 방법을 안내합니다.
#1. Retrofit 2 라이브러리 소개
Retrofit 2는 안드로이드 앱에서 서버와의 HTTP 통신을 쉽게 다룰 수 있도록 도와주는 라이브러리입니다.
Retrofit 2를 사용하면 네트워크 요청을 간편하게 생성하고 응답을 처리할 수 있습니다.
#2. 의존성 추가
안드로이드 프로젝트의 build.gradle 파일에 아래와 같이 Retrofit 2 의존성을 추가합니다.
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0' // JSON 파싱을 위한 Gson 컨버터
}
#3. API 인터페이스 생성
Retrofit 2를 사용하기 위해 API와 통신을 담당할 인터페이스를 생성합니다.
public interface ApiService {
@GET("endpoint")
Call<ResponseBody> fetchData(); // 네트워크 요청 메서드
}
#4. Retrofit 인스턴스 생성
앱의 Application 클래스나 액티비티에서 Retrofit 인스턴스를 생성합니다.
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/") // 기본 URL 설정
.addConverterFactory(GsonConverterFactory.create()) // Gson 컨버터 추가
.build();
ApiService apiService = retrofit.create(ApiService.class); // 인터페이스와 연결
#5. 네트워크 요청 보내기
Retrofit을 이용하여 서버로 네트워크 요청을 보내고 응답을 처리합니다.
Call<ResponseBody> call = apiService.fetchData(); // 네트워크 요청 생성
call.enqueue(new Callback<ResponseBody>() {
@Override
public void onResponse(Call<ResponseBody> call, Response<ResponseBody> response) {
if (response.isSuccessful()) {
// 성공적인 응답 처리
ResponseBody responseBody = response.body();
// TODO: responseBody 활용
} else {
// 응답이 실패한 경우 처리
}
}
@Override
public void onFailure(Call<ResponseBody> call, Throwable t) {
// 네트워크 요청 실패 처리
}
});
이렇게 Retrofit 2를 활용하여 안드로이드 앱에서 외부 서버와의 네트워크 통신을 구현할 수 있습니다. Retrofit 2를 사용하면 네트워크 요청과 응답 처리가 간편하며 효율적으로 이루어집니다. 외부 서버와 원활한 통신을 위해 Retrofit 2를 활용하여 안드로이드 앱을 개발해보세요.
레트로핏!!! 학교에서 프로젝트 실습에서 아주 질리도록 썼던 기억이 있네요..ㅎㅎ 좋은 정보 공유 감사합니다😄